Draft for Information Only
Content
Element Object Examples Element Object Property Properties included from Slotable
Event handlers
Element Object Method Element Object Event Clipboard events
Composition events
Focus events
Fullscreen events
Keyboard events
Mouse events
Touch events
Others Misc. Source/Reference
Element Object
The Element object is used to represent an element of the DOM tree of any web document loaded in the user agent. In general, element object is only related to the container of the DOM document tree. The element object only has methods and properties common to all kinds of elements. More specific classes inherit from Element. For example, the HTMLElement interface is the base interface for HTML elements, while the SVGElement interface is the basis for all SVG elements.
ExamplesExamples of document object ASP.NET Code Input:<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>Sample Page</title>
<meta http-equiv="Content-Type" content="text/html;charset=utf-8">
</head>
<body>
<%Response.Write("<p>Results on "& Request.ServerVariables("SERVER_SOFTWARE") & " .net: " & System.Environment.Version.ToString & " " & ScriptEngine & " Version " & ScriptEngineMajorVersion & "." & ScriptEngineMinorVersion & "</p>")%>
<!-- comment -->
<DIV>
<TABLE>
<TR>
<TD>Table cell 1
</TD>
</TR>
<TR>
<TD>Table cell 2
</TD>
</TR>
</TABLE>
</DIV>
<PRE id="demo">
</PRE>
<script type = "text/javascript">
var mynode = document.getElementsByTagName("TR")[0].childNodes
document.write("TR childNotes[1] nodeName: " + mynode[1].nodeName + "<br>");
document.write("TR childNotes[1] textContent: " + mynode[1].textContent + "<br>");
document.write("TR childNotes[1] firstChild.nodeName: " + mynode[1].firstChild.nodeName + "<br>");
document.write("TR childNotes[1] firstChild.textContent: " + mynode[1].firstChild.textContent + "<br>");
</script>
</body>
</html>
HTTP Response Output:<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>Sample Page</title>
<meta http-equiv="Content-Type" content="text/html;charset=utf-8">
</head>
<body>
<p>Results on Microsoft-IIS/8.5 .net: 2.0.50727.8000 VB Version 8.0</p>
<!-- comment -->
<DIV>
<TABLE>
<TR>
<TD>Table cell 1
</TD>
</TR>
<TR>
<TD>Table cell 2
</TD>
</TR>
</TABLE>
</DIV>
<PRE id="demo">
</PRE>
<script type = "text/javascript">
var mynode = document.getElementsByTagName("TR")[0].childNodes
document.write("TR childNotes[1] nodeName: " + mynode[1].nodeName + "<br>");
document.write("TR childNotes[1] textContent: " + mynode[1].textContent + "<br>");
document.write("TR childNotes[1] firstChild.nodeName: " + mynode[1].firstChild.nodeName + "<br>");
document.write("TR childNotes[1] firstChild.textContent: " + mynode[1].firstChild.textContent + "<br>");
</script>
</body>
</html>
HTML Web Page Embedded Output:
Element Object Property
Some typical Document object properties are.
Inherits properties from its parent interface, Node, and by extension that interface's parent, EventTarget. It implements the properties of ParentNode, ChildNode, NonDocumentTypeChildNode, and Animatable.
- Element.attributes: Read only. Returns a NamedNodeMap object containing the assigned attributes of the corresponding HTML element.
- Element.classList: Read only. Returns a DOMTokenList containing the list of class attributes.
- Element.className: Is a DOMString representing the class of the element.
- Element.clientHeight: Read only. Returns a Number representing the inner height of the element.
- Element.clientLeft: Read only. Returns a Number representing the width of the left border of the element.
- Element.clientTop: Read only. Returns a Number representing the width of the top border of the element.
- Element.clientWidth: Read only. Returns a Number representing the inner width of the element.
- Element.computedName: Read only. Returns a DOMString containing the label exposed to accessibility.
- Element.computedRole: Read only. Returns a DOMString containing the ARIA role that has been applied to a particular element.
- Element.id: Is a DOMString representing the id of the element.
- Element.innerHTML: Is a DOMString representing the markup of the element's content.
- Element.localName: Read only. A DOMString representing the local part of the qualified name of the element.
- Element.namespaceURI: Read only. The namespace URI of the element, ornull if it is no namespace.
Note: In Firefox 3.5 and earlier, HTML elements are in no namespace. In later versions, HTML elements are in the http://www.w3.org/1999/xhtml namespace in both HTML and XML trees.
- NonDocumentTypeChildNode.nextElementSibling: Read only. Is an Element, the element immediately following the given one in the tree, ornull if there's no sibling node.
- Element.outerHTML: Is a DOMString representing the markup of the element including its content. When used as a setter, replaces the element with nodes parsed from the given string.
- Element.prefix: Read only. A DOMString representing the namespace prefix of the element, ornull if no prefix is specified.
- NonDocumentTypeChildNode.previousElementSibling: Read only. Is a Element, the element immediately preceding the given one in the tree, ornull if there is no sibling element.
- Element.scrollHeight: Read only. Returns a Number representing the scroll view height of an element.
- Element.scrollLeft: Is a Number representing the left scroll offset of the element.
- Element.scrollLeftMax: This API has not been standardized. Read only. Returns a Number representing the maximum left scroll offset possible for the element.
- Element.scrollTop: A Number representing number of pixels the top of the document is scrolled vertically.
- Element.scrollTopMax: This API has not been standardized. Read only. Returns a Number representing the maximum top scroll offset possible for the element.
- Element.scrollWidth: Read only. Returns a Number representing the scroll view width of the element.
- Element.shadowRoot: Read only. Returns the open shadow root that is hosted by the element, or null if no open shadow root is present.
- Element.openOrClosedShadowRoot: This API has not been standardized. Read only. Returns the shadow root that is hosted by the element, regardless if its open or closed. Available only to WebExtensions.
- Element.slot: This is an experimental API that should not be used in production code. Returns the name of the shadow DOM slot the element is inserted in.
- Element.tabStop: This API has not been standardized. Is a Boolean indicating if the element can receive input focus via the tab key.
- Element.tagName: Read only. Returns a String with the name of the tag for the given element.
- Element.undoManager: This is an experimental API that should not be used in production code. Read only. Returns the UndoManager associated with the element.
- Element.undoScope: This is an experimental API that should not be used in production code. Is a Boolean indicating if the element is an undo scope host, or not.
Note: DOM Level 3 definednamespaceURI,localName andprefix on the Node interface. In DOM4 they were moved toElement.
This change is implemented in Chrome since version 46.0 and Firefox since version 48.0.
Properties included from Slotable
TheElement interface includes the following property, defined on the Slotable mixin.
- Slotable.assignedSlot: Read only. Returns a HTMLSlotElement representing the <slot> the node is inserted in.
Event handlers
- Element.onfullscreenchange: An event handler for the fullscreenchange event, which is sent when the element enters or exits full-screen mode. This can be used to watch both for successful expected transitions, but also to watch for unexpected changes, such as when your app is backgrounded.
- Element.onfullscreenerror: An event handler for the fullscreenerror event, which is sent when an error occurs while attempting to change into full-screen mode.
Element Object Method
Some typical Document object methods are.
Inherits methods from its parents Node, and its own parent, EventTarget, and implements those of ParentNode,
ChildNode, NonDocumentTypeChildNode, and Animatable.
- EventTarget.addEventListener(): Registers an event handler to a specific event type on the element.
- Element.attachShadow(): Attatches a shadow DOM tree to the specified element and returns a reference to its ShadowRoot.
- Element.animate(): This is an experimental API that should not be used in production code. A shortcut method to create and run an animation on an element. Returns the created Animation object instance.
- Element.closest(): This is an experimental API that should not be used in production code. Returns the Element which is the closest ancestor of the current element (or the current element itself) which matches the selectors given in parameter.
- Element.createShadowRoot(): This API has not been standardized. This deprecated API should no longer be used, but will probably still work. Creates a shadow DOM on on the element, turning it into a shadow host. Returns a ShadowRoot.
- Element.computedStyleMap(): This is an experimental API that should not be used in production code. Returns a StylePropertyMapReadOnly interface which provides a read-only representation of a CSS declaration block that is an alternative to CSSStyleDeclaration.
- EventTarget.dispatchEvent(): Dispatches an event to this node in the DOM and returns a Boolean that indicates whether no handler canceled the event.
- Element.getAnimations(): This is an experimental API that should not be used in production code. Returns an array of Animation objects currently active on the element.
- Element.getAttribute(): Retrieves the value of the named attribute from the current node and returns it as an Object.
- Element.getAttributeNames(): Returns an array of attribute names from the current element.
- Element.getAttributeNS(): Retrieves the value of the attribute with the specified name and namespace, from the current node and returns it as an Object.
- Element.getAttributeNode(): This is an obsolete API and is no longer guaranteed to work. Retrieves the node representation of the named attribute from the current node and returns it as an Attr.
- Element.getAttributeNodeNS(): This is an obsolete API and is no longer guaranteed to work. Retrieves the node representation of the attribute with the specified name and namespace, from the current node and returns it as an Attr.
- Element.getBoundingClientRect(): Returns the size of an element and its position relative to the viewport.
- Element.getClientRects(): Returns a collection of rectangles that indicate the bounding rectangles for each line of text in a client.
- Element.getElementsByClassName(): Returns a live HTMLCollection that contains all descendants of the current element that possess the list of classes given in the parameter.
- Element.getElementsByTagName(): Returns a live HTMLCollection containing all descendant elements, of a particular tag name, from the current element.
- Element.getElementsByTagNameNS(): Returns a live HTMLCollection containing all descendant elements, of a particular tag name and namespace, from the current element.
- Element.hasAttribute(): Returns a Boolean indicating if the element has the specified attribute or not.
- Element.hasAttributeNS(): Returns a Boolean indicating if the element has the specified attribute, in the specified namespace, or not.
- Element.hasAttributes(): Returns a Boolean indicating if the element has one or more HTML attributes present.
- Element.hasPointerCapture(): Indicates whether the element on which it is invoked has pointer capture for the pointer identified by the given pointer ID.
- Element.insertAdjacentElement(): Inserts a given element node at a given position relative to the element it is invoked upon.
- Element.insertAdjacentHTML(): Parses the text as HTML or XML and inserts the resulting nodes into the tree in the position given.
- Element.insertAdjacentText(): Inserts a given text node at a given position relative to the element it is invoked upon.
- Element.matches(): This is an experimental API that should not be used in production code. Returns a Boolean indicating whether or not the element would be selected by the specified selector string.
- Element.querySelector(): Returns the first Node which matches the specified selector string relative to the element.
- Element.querySelectorAll(): Returns a NodeList of nodes which match the specified selector string relative to the element.
- Element.releasePointerCapture(): Releases (stops) pointer capture that was previously set for a specific pointer event.
- ChildNode.remove(): This is an experimental API that should not be used in production code. Removes the element from the children list of its parent.
- Element.removeAttribute(): Removes the named attribute from the current node.
- Element.removeAttributeNS(): Removes the attribute with the specified name and namespace, from the current node.
- Element.removeAttributeNode(): This is an obsolete API and is no longer guaranteed to work. Removes the node representation of the named attribute from the current node.
- EventTarget.removeEventListener(): Removes an event listener from the element.
- Element.requestFullscreen(): This is an experimental API that should not be used in production code. Asynchronously asks the browser to make the element full-screen.
- Element.requestPointerLock(): This is an experimental API that should not be used in production code. Allows to asynchronously ask for the pointer to be locked on the given element.
- Element.scroll(): Scrolls to a particular set of coordinates inside a given element.
- Element.scrollBy(): Scrolls an element by the given amount.
- Element.scrollIntoView(): This is an experimental API that should not be used in production code. Scrolls the page until the element gets into the view.
- Element.scrollTo(): Scrolls to a particular set of coordinates inside a given element.
- Element.setAttribute(): Sets the value of a named attribute of the current node.
- Element.setAttributeNS(): Sets the value of the attribute with the specified name and namespace, from the current node.
- Element.setAttributeNode(): This is an obsolete API and is no longer guaranteed to work. Sets the node representation of the named attribute from the current node.
- Element.setAttributeNodeNS(): This is an obsolete API and is no longer guaranteed to work. Sets the node representation of the attribute with the specified name and namespace, from the current node.
- Element.setCapture(): This API has not been standardized. Sets up mouse event capture, redirecting all mouse events to this element.
- Element.setPointerCapture(): Designates a specific element as the capture target of future pointer events.
- Element.toggleAttribute(): Toggles a boolean attribute, removing it if it is present and adding it if it is not present, on the specified element.
Element Object Event
Some typical Document object events are.
Listen to these events usingaddEventListener() or by assigning an event listener to theon eventname property of this interface.
- cancel: Fires on a <dialog> when the user instructs the browser that they wish to dismiss the current open dialog. For example, the browser might fire this event when the user presses the Esc key or clicks a "Close dialog" button which is part of the browser's UI.
Also available via the oncancel property.
- error Fired when when a resource failed to load, or can't be used. For example, if a script has an execution error or an image can't be found or is invalid.
Also available via the onerror property.
- scroll: Fired when the document view or an element has been scrolled.
Also available via the onscroll property.
- select: Fired when some text has been selected.
Also available via the onselect property.
- show: Fired when a contextmenu event was fired on/bubbled to an element that has a contextmenu attribute. This deprecated API should no longer be used, but will probably still work.
Also available via the onshow property.
- wheel: Fired when the user rotates a wheel button on a pointing device (typically a mouse).
Also available via the onwheel property.
Clipboard events
- copy: Fired when the user initiates a copy action through the browser's user interface.
Also available via the oncopy property.
- cut: Fired when the user initiates a cut action through the browser's user interface.
Also available via the oncut property.
- paste: Fired when the user initiates a paste action through the browser's user interface.
Also available via the onpaste property.
Composition events
- compositionend: Fired when a text composition system such as an input method editor completes or cancels the current composition session.
- compositionstart: Fired when a text composition system such as an input method editor starts a new composition session.
- compositionupdate: Fired when a new character is received in the context of a text composition session controlled by a text composition system such as an input method editor.
Focus events
- blur: Fired when an element has lost focus.
Also available via the onblur property.
- focus: Fired when an element has gained focus.
Also available via the onfocus property
- focusin: Fired when an element is about to gain focus.
- focusout: Fired when an element is about to lose focus.
Fullscreen events
- fullscreenchange: Sent to an Element when it transitions into or out of full-screen mode.
Also available via the onfullscreenchange property.
- fullscreenerror: Sent to anElement if an error occurs while attempting to switch it into or out of full-screen mode.
Also available via the onfullscreenerror property.
Keyboard events
- keydown: Fired when a key is pressed.
Also available via the onkeydown property.
- keypress: Fired when a key that produces a character value is pressed down. This deprecated API should no longer be used, but will probably still work.
Also available via the onkeypress property.
- keyup: Fired when a key is released.
Also available via the onkeyup property.
Mouse events
- Activate: Occurs when an element is activated, for instance, through a mouse click or a keypress.
Also available via the onactivate property.
- auxclick: Fired when a non-primary pointing device button (e.g., any mouse button other than the left button) has been pressed and released on an element.
Also available via the onauxclick property.
- click: Fired when a pointing device button (e.g., a mouse's primary button) is pressed and released on a single element.
Also available via the onclick property.
- contextmenu: Fired when the user attempts to open a context menu.
Also available via the oncontextmenu property.
- dblclick: Fired when a pointing device button (e.g., a mouse's primary button) is clicked twice on a single element.
Also available via the ondblclick property.
- mousedown: Fired when a pointing device button is pressed on an element.
Also available via the onmousedown property.
- mouseenter: Fired when a pointing device (usually a mouse) is moved over the element that has the listener attached.
Also available via the onmouseenter property.
- mouseleave: Fired when the pointer of a pointing device (usually a mouse) is moved out of an element that has the listener attached to it.
Also available via the onmouseleave property.
- mousemove: Fired when a pointing device (usually a mouse) is moved while over an element.
Also available via the onmousemove property.
- mouseout: Fired when a pointing device (usually a mouse) is moved off the element to which the listener is attached or off one of its children.
Also available via the onmouseout property.
- mouseover: Fired when a pointing device is moved onto the element to which the listener is attached or onto one of its children.
Also available via the onmouseover property.
- mouseup: Fired when a pointing device button is released on an element.
Also available via the onmouseup property.
- webkitmouseforcechanged: Fired each time the amount of pressure changes on the trackpadtouchscreen.
- webkitmouseforcedown: Fired after the mousedown event as soon as sufficient pressure has been applied to qualify as a "force click".
- webkitmouseforcewillbegin: Fired before the mousedown event.
- webkitmouseforceup: Fired after the webkitmouseforcedown event as soon as the pressure has been reduced sufficiently to end the "force click".
Touch events
- touchcancel: Fired when one or more touch points have been disrupted in an implementation-specific manner (for example, too many touch points are created).
Also available via the ontouchcancel property.
- touchend: Fired when one or more touch points are removed from the touch surface.
Also available via the ontouchend property
- touchmove: Fired when one or more touch points are moved along the touch surface.
Also available via the ontouchmove property
- touchstart: Fired when one or more touch points are placed on the touch surface.
Also available via the ontouchstart property
Others Misc.
- accessKey: Sets or returns the accesskey attribute of an element
- appendChild(): Adds a new child node, to an element, as the last child node
- childElementCount: Returns the number of child elements an element has
- childNodes: Returns a collection of an element's child nodes (including text and comment nodes)
- children: Returns a collection of an element's child element (excluding text and comment nodes)
- cloneNode(): Clones an element
- compareDocumentPosition(): Compares the document position of two elements
- contains(): Returns true if a node is a descendant of a node, otherwise false
- contentEditable: Sets or returns whether the content of an element is editable or not
- dir: Sets or returns the value of the dir attribute of an element
- exitFullscreen(): Cancels an element in fullscreen mode
- firstChild: Returns the first child node of an element
- firstElementChild: Returns the first child element of an element
- hasChildNodes(): Returns true if an element has any child nodes, otherwise false
- innerText: Sets or returns the text content of a node and its descendants
- insertBefore(): Inserts a new child node before a specified, existing, child node
- isContentEditable: Returns true if the content of an element is editable, otherwise false
- isDefaultNamespace(): Returns true if a specified namespaceURI is the default, otherwise false
- isEqualNode(): Checks if two elements are equal
- isSameNode(): Checks if two elements are the same node
- isSupported(): Returns true if a specified feature is supported on the element
- lang: Sets or returns the value of the lang attribute of an element
- lastChild: Returns the last child node of an element
- lastElementChild: Returns the last child element of an element
- nextSibling: Returns the next node at the same node tree level
- nodeName: Returns the name of a node
- nodeType: Returns the node type of a node
- nodeValue: Sets or returns the value of a node
- normalize(): Joins adjacent text nodes and removes empty text nodes in an element
- offsetHeight: Returns the height of an element, including padding, border and scrollbar
- offsetWidth: Returns the width of an element, including padding, border and scrollbar
- offsetLeft: Returns the horizontal offset position of an element
- offsetParent: Returns the offset container of an element
- offsetTop: Returns the vertical offset position of an element
- ownerDocument: Returns the root element (document object) for an element
- parentNode: Returns the parent node of an element
- parentElement: Returns the parent element node of an element
- previousSibling: Returns the previous node at the same node tree level
- removeChild(): Removes a child node from an element
- replaceChild(): Replaces a child node in an element
- style: Sets or returns the value of the style attribute of an element
- tabIndex: Sets or returns the value of the tabindex attribute of an element
- textContent: Sets or returns the textual content of a node and its descendants
- title: Sets or returns the value of the title attribute of an element
- toString(): Converts an element to a string
Source/Reference
- https://developer.mozilla.org/en-US/docs/Web/API/Element
- https://www.w3schools.com/jsref/dom_obj_all.asp
©sideway
ID: 190500023 Last Updated: 5/23/2019 Revision: 0
|
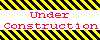 |