Draft for Information Only
Content
Python Functions Types of Built-in Functions Class Functions Built-In Types Numeric Types Sequence Types Set Types Mapping Types Other Built-in Types System Functions Data Functions Conversion Functions Mathematical Functions Source and Reference
Python Functions
The Python interpreter has a number of functions and types built into it that are always available.
Types of Built-in Functions
Class Functions
Built-In Types
Numeric Types
class int([x]) class int(x, base=10)Return an integer object.
class float([x])Return a formatted representation
class complex([real[, imag]])Return a complex number with the value real + imag*1j
class bool([x])Return a Boolean value, i.e. one of True or False.
Sequence Types
class list([iterable])Return a mutable sequence.
class tuple([iterable])Return an immutable sequence.
class range(stop) class range(start, stop[, step])Return an immutable sequence
class str(object='') class str(object=b'', encoding='utf-8', errors='strict')Return a string object.
class bytearray([source[, encoding[, errors]]])Return a mutable sequence of bytes.
class bytes([source[, encoding[, errors]]])Return an immutable sequence of bytes.
class memoryview(obj)Return a memory view object
Set Types
class set([iterable])Return a new set object.
class frozenset([iterable])Return a frozenset object.
Mapping Types
class dict(**kwarg) class dict(mapping, **kwarg) class dict(iterable, **kwarg)Return a new dictionary object.
Other Built-in Types
class objectReturn a featureless object.
class propertyReturn a property attribute.
class type(object) class type(name, bases, dict)Return the type of an object.
class slice(stop) class slice(start, stop[, step])Return a slice object representing the set of indices specified by range(start, stop, step).
System Functions
breakpoint(*args, **kws)Return a system break.
@classmethodTransform a method into a class method.
compile(source, filename, mode, flags=0, dont_inherit=False, optimize=-1)Compile the source into a code or AST object.
delattr(object, name)Delete the named attribute, provided the object allows it.
dir([object])Return the list of valid attributes.
eval(expression[, globals[, locals]])Evaluate the expression.
exec(object[, globals[, locals]])Execute the object.
getattr(object, name[, default])Return the value of the named attribute of object.
globals()Return a dictionary representing the current global symbol table.
hasattr(object, name)Return a bool to indicate the name is one of the object's attributes.
hash(object)Return the hash value of the object, if it has one.
help([object])Invoke the built-in help system.
id(object)Return the integer identity of an object.
input([prompt])Read a line from input.
locals()Update and return a dictionary representing the current local symbol table.
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)Open file and return a corresponding file object.
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)Print objects to the text stream file.
repr(object)Return a string containing a printable representation of an object.
setattr(object, name, value)Assign the value to the attribute, provided the object allows it.
@staticmethodTransform a method into a static method.
super([type[, object-or-type]])Return a proxy object that delegates method calls to a parent or sibling class of type.
vars([object])Return the __dict__ attribute for a module, class, instance, or any other object with a __dict__ attribute.
__import__(name, globals=None, locals=None, fromlist=(), level=0)The function imports the module name, potentially using the given globals and locals to determine how to interpret the name in a package context. This function is invoked by the import statement.
Data Functions
Conversion Functions
ascii(object)Return a string containing a printable representation of an object, but escape the non-ASCII characters in the string returned by repr() using \x, \u or \U escapes.
bin(x)Convert an integer number to a binary string prefixed with “0b”.
chr(i)Return the string representing a character whose Unicode code point is the integer i.
enumerate(iterable, start=0)Return an enumerate object. iterable must be a sequence, an iterator, or some other object which supports iteration.
filter(function, iterable)Return an iterator from those elements of iterable for which function returns true.
format(value[, format_spec])Convert a value to a “formatted” representation, as controlled by format_spec.
hex(x)Convert an integer number to a lowercase hexadecimal string prefixed with “0x”.
iter(object[, sentinel])Return an iterator object.
len(s)Return the length (the number of items) of an object.
map(function, iterable, ...)Return an iterator that applies function to every item of iterable, yielding the results.
max(iterable, *[, key, default])
max(arg1, arg2, *args[, key])Return the largest item in an iterable or the largest of two or more arguments.
min(iterable, *[, key, default])
min(arg1, arg2, *args[, key])Return the smallest item in an iterable or the smallest of two or more arguments.
next(iterator[, default])Retrieve the next item from the iterator by calling its __next__() method.
oct(x)Convert an integer number to an octal string prefixed with “0o”.
ord(c)Given a string representing one Unicode character, return an integer representing the Unicode code point of that character.
reversed(seq)Return a reverse iterator. seq must be an object which has a __reversed__() method or supports the sequence protocol (the __len__() method and the __getitem__() method with integer arguments starting at 0).
sorted(iterable, *, key=None, reverse=False)Return a sorted list from the items in iterable.
zip(*iterables)Returns an iterator of tuples, where the i-th tuple contains the i-th element from each of the argument sequences or iterables.
Mathematical Functions
abs(x)Return the absolute value of a number.
all(iterable)Return True if all elements of the iterable are true (or if the iterable is empty).
any(iterable)Return True if any element of the iterable is true. If the iterable is empty, return False.
callable(object)Return True if the object argument appears callable, False if not. If this returns True, it is still possible that a call fails, but if it is False, calling object will never succeed.
divmod(a, b)Take two (non complex) numbers as arguments and return a pair of numbers consisting of their quotient and remainder when using integer division. With mixed operand types, the rules for binary arithmetic operators apply.
isinstance(object, classinfo)Return True if the object argument is an instance of the classinfo argument, or of a (direct, indirect or virtual) subclass thereof. If object is not an object of the given type, the function always returns False.
issubclass(class, classinfo)Return True if class is a subclass (direct, indirect or virtual) of classinfo.
pow(base, exp[, mod])Return base to the power exp; if mod is present, return base to the power exp, modulo mod (computed more efficiently than pow(base, exp) % mod).
round(number[, ndigits])Return number rounded to ndigits precision after the decimal point.
sum(iterable, /, start=0)Sums start and the items of an iterable from left to right and returns the total.
Source and Reference
©sideway
ID: 210500003 Last Updated: 5/3/2021 Revision: 0
|
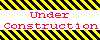 |