hash, sum, super, idlen, max, min, next, sumdelattr, dir, getattr, globals, locals, setattr, varsascii, format, reprinput, open, print __import__breakpoint, @classmethod, compile, eval, exec, help, @staticmethod
Draft for Information Only
Content
Python Built-in Conversion Functions breakpoint() Parameters Remarks @classmethod Parameters Remarks compile() Parameters Remarks eval() Parameters Remarks exec() Parameters Remarks help() Parameters Remarks @staticmethod Parameters Remarks Source and Reference
Python Built-in Conversion Functions
The Python interpreter has some built-in conversion functions.
breakpoint()
breakpoint(*args, **kws)
Parameters
breakpoint()to drop into the debugger at the call site.
*argsto specify the arugments
**kwsto specify the keyword arugments
Remarks
- Specifically, it calls
sys.breakpointhook(), passing args and kws straight through.
- By default,
sys.breakpointhook() calls pdb.set_trace() expecting no arguments.
- In this case, it is purely a convenience function so you don’t have to explicitly import pdb or type as much code to enter the debugger. However, sys.breakpointhook() can be set to some other function and breakpoint() will automatically call that, allowing you to drop into the debugger of choice.
- Raises an auditing event builtins.breakpoint with argument breakpointhook.
@classmethod
@classmethod
Parameters
type()to transform a method into a class method.
Remarks
- A class method receives the class as implicit first argument, just like an instance method receives the instance.
- To declare a class method, use this idiom:
class C:
@classmethod
def f(cls, arg1, arg2, ...): ...
- The @classmethod form is a function decorator
- A class method can be called either on the class (such as C.f()) or on an instance (such as C().f()).
- The instance is ignored except for its class. If a class method is called for a derived class, the derived class object is passed as the implied first argument.
- Class methods are different than C++ or Java static methods. If you want those, see staticmethod()
compile()
compile(source, filename, mode, flags=0, dont_inherit=False, optimize=-1)
Parameters
compile()to compile the specified source into a code or AST object.
sourceto specify the source to be returned from.
filenameto specify the file from which the code was read
modeto specify what kind of code must be compiled
flagsto control which future statements affect the compilation of source.
dont_inheritto control which future statements affect the compilation of source.
optimizeto specifies the optimization level of the compiler.
Remarks
- Code objects can be executed by
exec() or eval()
source can either be a normal string, a byte string, or an AST object
filenamepass: some recognizable value if it wasn't read from a file ('<string>" is commonly used).
mode can be 'exec' if source consists of a sequence of statements; 'eval' if it consists of a single expression; or 'single' if it consists of a single interactive statement (in the latter case, expression statements that evaluate to something othe than None will be printed.
- If neither
flags nor dont_inherit is present (or both are zero), the code is compiled with those future statements that are in effect in the code that is calling compile()
- If
flags is given and dont_inherit is not (or is zero) then the future statements specified by the flags argument are used in addition to those that would be used anyway.
- If
dont_inherit is a non-zero integer then the flags argument is it -- the future statements in effect around the call to compile are ignored.
- Future statements are specified by bits which can be bitwise ORed together to specify multiple statements. The bitfield required to specify a given feature can be found as the
compiler_flag attribute on the _Feature instance in the __future__ module.
- The optional argument
flags also controls whether the compiled source is allowed to contain top-level await, async for and async with. When the bit ast.PyCF_ALLOW_TOP_LEVEL_AWAIT is set, the return code object has CO_COROUTINE set in co_code, and can be interactively executed via await eval(code_object)
- The default value of
optimize is -1.
optimize selects the optimization level of the interpreter as given by -o options. Explicit levels are 0, (no optimization; __debug__ is true), 1 (asserts are removed, __debug__ is false), or 2 (docstrings are removed too).
- If the compiled source is invalid,
compile raises SyntaxError
- If the source contains null bytes,
compile raises ValueError
- Raises an auditing event
compile with arguments source and filename. This event may also be raised by implicit compilation.
- When compiling a string with multi-line code in
'single' or 'eval' mode, input must be terminated by at least one newline character. This is to facilitate detection of incomplete and complete statements in the code module
- It is possible to crash the Python interpreter with a sufficiently large/complex string when compiling to an AST object due to stack depth limitations in Python’s AST compiler.
eval()
eval(expression[, globals[, locals]])
Parameters
eval()to return the result of the evaluated expression.
expressionto specify the expression to be evaluated
[globals]optional, to specify the globals to be used
[locals]optional, to specify the locals to be used
Remarks
globals must be a dictionary.
localscan be any mapping object.
expression is parsed and evaluated as a Python expression using the globals and locals dictionaries as global and local namespace.
- If the
globals dictionary is present and does not contain a value for the key __builtins__, a reference to the dictionary of the built-in module builtins is inserted under that key before expression is parsed. This means that expression normally has full access to the standard builtins module and restricted environments are propagated. If the locals dictionary is omitted it defaults to the globals dictionary. If both dictinaries are omitted, the expression is executed with the globals and locals in the environment where eval() is called.
eval() does not have access to the nested scopes (non-locals) in the enclosing environment.
- The return value is the result of the evaluated expression. Syntax errors are reported as exceptions.
- This function can also be used to execute arbitrary code objects (such as those created by
compile(). In this case pass a code object instead of a string. If the code object has been compiled with 'exec' as the mode argument, eval()'s return value will be None.
- Dynamic execution of statements is supported by the
exec() function. The globals() and locals() functions returns the current global and local dictionary, respectively, which may be useful to pass around for use by eval() or exec().
- See
ast.literal_eval() for a function that can safely evaluate strings with expressions containing only literals.
- Raises an auditing event
exec with the code object as the argument. Code compilation events may also be raised.
exec()
exec(object[, globals[, locals]])
Parameters
exec()to
objectto specify the code to be executed
[globals]optional, to specify the globals to be used
[locals]optional, to specify the locals to be used
Remarks
- To supports dynamic execution of Python code.
object must be either a string or a code object.
- If
object is a string, the string is parsed as a suite of Python statements which is then executed (unless a syntax error occurs).
- If
object is a code object, it is simply executed.
- In all cases, the code that's executed is expected to be valid as file input.
- Be aware that the
return and yield statements may not be used outside of function definitions even within the context of code passed to the exec() function.
- The return value is
None.
- In all cases, if the optional parts are omitted, the code is executed in the current scope. If only
globals is provided, it must be a dictionary (and not a subclass of dictionary), which will be used for both the global and the local variables. If globals and locals are given, they are used for the global and local variables, respectively. If provided, locals can be any mapping object. Remember that at module level, globals and locals are the same dictionary. If exec gets two separate objects as globals and locals, the code will be executed as if it were embedded in a class definition.
- If the
globals dictionary does not contain a value for the key __builtins__, a reference to the dictionary of the built-in module builtins is inserted under that key. That way you can control what builtins are available to the executed code by inserting your own __builtins__ dictionary into globals before passing it to exec().
- Raises an auditing event
exec with the code object as the argument. Code compilation events may also be raised.
- The built-in functions
globals() and locals() return the current global and local dictionary, respectively, which may be useful to pass around for use as the second and third argument to exec().
- The default
locals act as described for function locals() below: modifications to the default locals dictionary should not be attempted/ Pass an explicit locala dictionary if you need to see effects of the code on locals after function exec() returns.
help()
help([object])
Parameters
help()to invoke the built-in help system.
[object]optional, to specify the object to be returned from
Remarks
help() is intended fro interactive use.
- If
object is omitted, the interactive help system starts on the interpreter console.
- if
object is a string, then the string is looked up as the name of a module, function, class, method, keyword, or documentation topic, and a help page is printed on the console.
- If the argument is any other kind of object, a help page on the object is generated.
- If a slash
/ appears in the parameter list of a function, when invoking help(), it means that the parameters prior to slash are positional-only.
help() is added to the built-in namespace by the site module.
@staticmethod
@staticmethod
Parameters
@staticmethodto transform a method into a static method.
Remarks
- A static method does not receive an implicit first argument. To declare a static method, use this idiom:
class C:
@staticmethod
def f(arg1, arg2, ...): ...
The @staticmethod form is a function decorator – see Function definitions for details.
A static method can be called either on the class (such as C.f()) or on an instance (such as C().f()).
Static methods in Python are similar to those found in Java or C++. Also see classmethod() for a variant that is useful for creating alternate class constructors.
Like all decorators, it is also possible to call staticmethod as a regular function and do something with its result. This is needed in some cases where you need a reference to a function from a class body and you want to avoid the automatic transformation to instance method. For these cases, use this idiom:
class C:
builtin_open = staticmethod(open)
Source and Reference
©sideway
ID: 201202902 Last Updated: 12/29/2020 Revision: 0
|
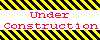 |