Draft for Information Only
Content
Manim Coordinate System Codes in Coordinate_systems.py Import Class CoordinateSystem() Configuration Functions Class Axes(VGroup, CoordinateSystem) Configuration Functions Class ThreeDAxes(Axes) Configuration Functions Class NumberPlane(Axes) Configuration Functions Class ComplexPlane(NumberPlane) Configuration Functions Tree Structure Source and Reference
Manim Coordinate System
The physical position of a Mobject object in Manim is based on a Cartesian rectangular coordinate system. Coordinate System VMobject objects are used to visualize the coordinate system of Scene object.
Codes in Coordinate_systems.py
Available codes defined in manimlib.mobject.coordinate_systems.py

Five classes, CoordinateSystem, Axes, ThreeDAxes, NumberPlane, and ComplexPlane are defined.
Import
The import defined in manimlib.mobject.coordinate_systems.py:
import numpy as np
import numbers
from manimlib.constants import *
from manimlib.mobject.functions import ParametricFunction
from manimlib.mobject.geometry import Arrow
from manimlib.mobject.geometry import Line
from manimlib.mobject.number_line import NumberLine
from manimlib.mobject.svg.tex_mobject import TexMobject
from manimlib.mobject.types.vectorized_mobject import VGroup
from manimlib.utils.config_ops import digest_config
from manimlib.utils.config_ops import merge_dicts_recursively
from manimlib.utils.simple_functions import binary_search
from manimlib.utils.space_ops import angle_of_vector
Class CoordinateSystem()
class manimlib.mobject.coordinate_systems.CoordinateSystem()version 19Dec2019
Configuration
CONFIG = {
"dimension": 2,
"x_min": -FRAME_X_RADIUS,
"x_max": FRAME_X_RADIUS,
"y_min": -FRAME_Y_RADIUS,
"y_max": FRAME_Y_RADIUS,
}
Functions
Functions defined in class CoordinateSystem are
- """Abstract class for Axes and NumberPlane"""
- def coords_to_point(self, *coords)
- def point_to_coords(self, point)
- def c2p(self, *coords)
- def p2c(self, point)
- def get_axes(self)
- def get_axis(self, index)
- def get_x_axis(self)
- def get_y_axis(self)
- def get_z_axis(self)
- def get_x_axis_label(self, label_tex, edge=RIGHT, direction=DL, **kwargs)
- def get_y_axis_label(self, label_tex, edge=UP, direction=DR, **kwargs)
- def get_axis_label(self, label_tex, axis, edge, direction, buff=MED_SMALL_BUFF)
- def get_axis_labels(self, x_label_tex="x", y_label_tex="y")
- def get_graph(self, function, **kwargs)
- def get_parametric_curve(self, function, **kwargs)
- def input_to_graph_point(self, x, graph)
Class Axes(VGroup, CoordinateSystem)
class manimlib.mobject.coordinate_systems.Axes(VGroup, CoordinateSystem)version 19Dec2019
Configuration
CONFIG = {
"number_line_config": {
"color": LIGHT_GREY,
"include_tip": True,
"exclude_zero_from_default_numbers": True,
},
"x_axis_config": {},
"y_axis_config": {
"label_direction": LEFT,
},
"center_point": ORIGIN,
}
Functions
Functions defined in class Axes are
- def __init__(self, **kwargs)
- def create_axis(self, min_val, max_val, axis_config)
- def coords_to_point(self, *coords)
- def c2p(self, *coords)
- def point_to_coords(self, point)
- def p2c(self, point)
- def get_axes(self)
- def get_coordinate_labels(self, x_vals=None, y_vals=None)
- def add_coordinates(self, x_vals=None, y_vals=None)
Class ThreeDAxes(Axes)
class manimlib.mobject.coordinate_systems.ThreeDAxes(Axes)version 19Dec2019
Configuration
CONFIG = {
"dimension": 3,
"x_min": -5.5,
"x_max": 5.5,
"y_min": -5.5,
"y_max": 5.5,
"z_axis_config": {},
"z_min": -3.5,
"z_max": 3.5,
"z_normal": DOWN,
"num_axis_pieces": 20,
"light_source": 9 * DOWN + 7 * LEFT + 10 * OUT,
}
Functions
Functions defined in class ThreeDAxes are
- def __init__(self, **kwargs)
- def add_3d_pieces(self)
- def set_axis_shading(self)
Class NumberPlane(Axes)
class manimlib.mobject.coordinate_systems.NumberPlane(Axes)version 19Dec2019
Configuration
CONFIG = {
"axis_config": {
"stroke_color": WHITE,
"stroke_width": 2,
"include_ticks": False,
"include_tip": False,
"line_to_number_buff": SMALL_BUFF,
"label_direction": DR,
"number_scale_val": 0.5,
},
"y_axis_config": {
"label_direction": DR,
},
"background_line_style": {
"stroke_color": BLUE_D,
"stroke_width": 2,
"stroke_opacity": 1,
},
# Defaults to a faded version of line_config
"faded_line_style": None,
"x_line_frequency": 1,
"y_line_frequency": 1,
"faded_line_ratio": 1,
"make_smooth_after_applying_functions": True,
}
Functions
Functions defined in class NumberPlane are
- def __init__(self, **kwargs)
- def init_background_lines(self)
- def get_lines(self)
- def get_lines_parallel_to_axis(self, axis1, axis2, freq, ratio)
- def get_center_point(self)
- def get_x_unit_size(self)
- def get_y_unit_size(self)
- def get_axes(self)
- def get_vector(self, coords, **kwargs)
- def prepare_for_nonlinear_transform(self, num_inserted_curves=50)
Class ComplexPlane(NumberPlane)
class manimlib.mobject.coordinate_systems.ComplexPlane(NumberPlane)version 19Dec2019
Configuration
CONFIG = {
"color": BLUE,
"line_frequency": 1,
}
Functions
Functions defined in class ComplexPlane are
- def number_to_point(self, number)
- def n2p(self, number)
- def point_to_number(self, point)
- def p2n(self, point)
- def get_default_coordinate_values(self)
- def get_coordinate_labels(self, *numbers, **kwargs)
- def add_coordinates(self, *numbers)
Tree Structure
Source and Reference
https://github.com/3b1b/manim 19Dec2019
©sideway
ID: 200301602 Last Updated: 3/16/2020 Revision: 0
|
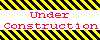 |