geometry.pyTipableVMobject, Arc, ArcBetweenPoints, CurvedArrow, CurvedDoubleArrow, CubicBezierAnnularSector, Sector, Circle, Ellipse, Annulus, Dot, SmallDotLine, DashedLine, TangentLine, Elbow, Arrow, DoubleArrow, VectorPolygon, RegularPolygon, Triangle, ArrowTip, Rectangle, Square, RoundedRectangle
Draft for Information Only
Content
Manim Geometry Codes in Geometry.py Import Constants Class TipableVMobject(VMobject) Configuration Functions Class Arc(TipableVMobject) Configuration Functions Class ArcBetweenPoints(Arc) Functions Class CurvedArrow(ArcBetweenPoints) Functions Class CurvedDoubleArrow(CurvedArrow) Functions Class Circle(Arc) Configuration Functions Class Dot(Circle) Configuration Functions Class SmallDot(Dot) Configuration Class Ellipse(Circle) Configuration Functions Class AnnularSector(Arc) Configuration Functions Class Sector(AnnularSector) Configuration Class Annulus(Circle) Configuration Functions Class Line(TipableVMobject) Configuration Functions Class DashedLine(Line) Configuration Functions Class TangentLine(Line) Configuration Functions Class Elbow(VMobject) Configuration Functions Class Arrow(Line) Configuration Functions Class Vector(Arrow) Configuration Functions Class DoubleArrow(Arrow) Functions Class CubicBezier(VMobject) Functions Class Polygon(VMobject) Configuration Functions Class RegularPolygon(Polygon) Configuration Functions Class Triangle(RegularPolygon) Functions Class ArrowTip(Triangle) Configuration Functions Class Rectangle(Polygon) Configuration Functions Class Square(Rectangle) Configuration Functions Class RoundedRectangle(Rectangle) Configuration Functions Source and Reference
Manim Geometry
The geometric VMobject object in Manim is mainly defined in geometry.py. Besides the base geometric elements, some common used geometric shapes are also defined
Codes in Geometry.py
Available codes defined in manimlib.mobject.geometry.py

Twenty-seven classes, TipableVMobject, Arc, ArcBetweenPoints, CurvedArrow, CurvedDoubleArrow, Circle, Dot, SmallDot, Ellipse, AnnularSector, Sector, Annulus, Line, DashedLine, TangentLine, Elbow, Arrow, Vector, DoubleArrow, CubicBezier, Polygon, RegularPolygon, Triangle, ArrowTip, Rectangle, Square and RoundedRectangle are defined.
Import
The import defined in manimlib.mobject.geometry.py:
import warnings
import numpy as np
from manimlib.constants import *
from manimlib.mobject.mobject import Mobject
from manimlib.mobject.types.vectorized_mobject import VGroup
from manimlib.mobject.types.vectorized_mobject import VMobject
from manimlib.mobject.types.vectorized_mobject import DashedVMobject
from manimlib.utils.config_ops import digest_config
from manimlib.utils.iterables import adjacent_n_tuples
from manimlib.utils.iterables import adjacent_pairs
from manimlib.utils.simple_functions import fdiv
from manimlib.utils.space_ops import angle_of_vector
from manimlib.utils.space_ops import angle_between_vectors
from manimlib.utils.space_ops import compass_directions
from manimlib.utils.space_ops import line_intersection
from manimlib.utils.space_ops import get_norm
from manimlib.utils.space_ops import normalize
from manimlib.utils.space_ops import rotate_vector
Constants
DEFAULT_DOT_RADIUS = 0.08
DEFAULT_SMALL_DOT_RADIUS = 0.04
DEFAULT_DASH_LENGTH = 0.05
DEFAULT_ARROW_TIP_LENGTH = 0.35
Class TipableVMobject(VMobject)
class manimlib.mobject.geometry.TipableVMobject(VMobject)version 19Dec2019
Configuration
CONFIG = {
"tip_length": DEFAULT_ARROW_TIP_LENGTH,
# TODO
"normal_vector": OUT,
"tip_style": {
"fill_opacity": 1,
"stroke_width": 0,
}
}
Functions
Functions defined in class CoordinateSystem are
- # Adding, Creating, Modifying tips
- def add_tip(self, tip_length=None, at_start=False)
- def create_tip(self, tip_length=None, at_start=False)
- def get_unpositioned_tip(self, tip_length=None)
- def position_tip(self, tip, at_start=False)
- def reset_endpoints_based_on_tip(self, tip, at_start)
- def asign_tip_attr(self, tip, at_start)
- # Checking for tips
- def has_tip(self)
- def has_start_tip(self)
- # Getters
- def pop_tips(self)
- def get_tips(self)
- def get_tip(self)
- def get_default_tip_length(self)
- def get_first_handle(self)
- def get_last_handle(self)
- def get_end(self)
- def get_start(self)
- def get_length(self)
Class Arc(TipableVMobject)
class manimlib.mobject.geometry.Arc(TipableVMobject)version 19Dec2019
Configuration
CONFIG = {
"radius": 1.0,
"num_components": 9,
"anchors_span_full_range": True,
"arc_center": ORIGIN,
}
Functions
Functions defined in class CoordinateSystem are
- def __init__(self, start_angle=0, angle=TAU / 4, **kwargs)
- def generate_points(self)
- def set_pre_positioned_points(self)
- def get_arc_center(self)
- def move_arc_center_to(self, point)
- def stop_angle(self)
Class ArcBetweenPoints(Arc)
class manimlib.mobject.geometry.ArcBetweenPoints(Arc)version 19Dec2019
Functions
Functions defined in class CoordinateSystem are
- def __init__(self, start, end, angle=TAU / 4, **kwargs)
Class CurvedArrow(ArcBetweenPoints)
class manimlib.mobject.geometry.CurvedArrow(ArcBetweenPoints)version 19Dec2019
Functions
Functions defined in class CoordinateSystem are
- def __init__(self, start_point, end_point, **kwargs)
Class CurvedDoubleArrow(CurvedArrow)
class manimlib.mobject.geometry.CurvedDoubleArrow(CurvedArrow)version 19Dec2019
Functions
Functions defined in class CoordinateSystem are
- def __init__(self, start_point, end_point, **kwargs)
Class Circle(Arc)
class manimlib.mobject.geometry.Circle(Arc)version 19Dec2019
Configuration
CONFIG = {
"color": RED,
"close_new_points": True,
"anchors_span_full_range": False
}
Functions
Functions defined in class CoordinateSystem are
- def __init__(self, **kwargs)
- def surround(self, mobject, dim_to_match=0, stretch=False, buffer_factor=1.2)
- def point_at_angle(self, angle)
Class Dot(Circle)
class manimlib.mobject.geometry.Dot(Circle)version 19Dec2019
Configuration
CONFIG = {
"radius": DEFAULT_DOT_RADIUS,
"stroke_width": 0,
"fill_opacity": 1.0,
"color": WHITE
}
Functions
- def __init__(self, point=ORIGIN, **kwargs)
Class SmallDot(Dot)
class manimlib.mobject.geometry.SmallDot(Dot)version 19Dec2019
Configuration
CONFIG = {
"radius": DEFAULT_SMALL_DOT_RADIUS,
}
Class Ellipse(Circle)
class manimlib.mobject.geometry.Ellipse(Circle)version 19Dec2019
Configuration
CONFIG = {
"width": 2,
"height": 1
}
Functions
- def __init__(self, **kwargs)
Class AnnularSector(Arc)
class manimlib.mobject.geometry.AnnularSector(Arc)version 19Dec2019
Configuration
CONFIG = {
"inner_radius": 1,
"outer_radius": 2,
"angle": TAU / 4,
"start_angle": 0,
"fill_opacity": 1,
"stroke_width": 0,
"color": WHITE,
}
Functions
- def generate_points(self)
Class Sector(AnnularSector)
class manimlib.mobject.geometry.Sector(AnnularSector)version 19Dec2019
Configuration
CONFIG = {
"outer_radius": 1,
"inner_radius": 0
}
Class Annulus(Circle)
class manimlib.mobject.geometry.Annulus(Circle)version 19Dec2019
Configuration
CONFIG = {
"inner_radius": 1,
"outer_radius": 2,
"fill_opacity": 1,
"stroke_width": 0,
"color": WHITE,
"mark_paths_closed": False,
}
Functions
- def generate_points(self)
Class Line(TipableVMobject)
class manimlib.mobject.geometry.Line(TipableVMobjectversion 19Dec2019
Configuration
CONFIG = {
"buff": 0,
"path_arc": None, # angle of arc specified here
}
Functions
- def __init__(self, start=LEFT, end=RIGHT, **kwargs)
- def generate_points(self)
- def set_path_arc(self, new_value)
- def account_for_buff(self)
- def set_start_and_end_attrs(self, start, end)
- def pointify(self, mob_or_point, direction=None)
- def put_start_and_end_on(self, start, end)
- def get_vector(self)
- def get_unit_vector(self)
- def get_angle(self)
- def get_slope(self)
- def set_angle(self, angle)
- def set_length(self, length)
- def set_opacity(self, opacity, family=True)
Class DashedLine(Line)
class manimlib.mobject.geometry.DashedLine(Line)version 19Dec2019
Configuration
CONFIG = {
"dash_length": DEFAULT_DASH_LENGTH,
"dash_spacing": None,
"positive_space_ratio": 0.5,
}
Functions
- def __init__(self, *args, **kwargs)
- def calculate_num_dashes(self, positive_space_ratio)
- def calculate_positive_space_ratio(self)
- def get_start(self)
- def get_end(self)
- def get_first_handle(self)
- def get_last_handle(self)
Class TangentLine(Line)
class manimlib.mobject.geometry.TangentLine(Line)version 19Dec2019
Configuration
CONFIG = {
"length": 1,
"d_alpha": 1e-6
}
Functions
- def __init__(self, vmob, alpha, **kwargs)
Class Elbow(VMobject)
class manimlib.mobject.geometry.Elbow(VMobject)version 19Dec2019
Configuration
CONFIG = {
"width": 0.2,
"angle": 0,
}
Functions
- def __init__(self, **kwargs)
Class Arrow(Line)
class manimlib.mobject.geometry.Arrow(Line)version 19Dec2019
Configuration
CONFIG = {
"stroke_width": 6,
"buff": MED_SMALL_BUFF,
"max_tip_length_to_length_ratio": 0.25,
"max_stroke_width_to_length_ratio": 5,
"preserve_tip_size_when_scaling": True,
}
Functions
- def __init__(self, *args, **kwargs)
- def scale(self, factor, **kwargs)
- def get_normal_vector(self)
- def reset_normal_vector(self)
- def get_default_tip_length(self)
- def set_stroke_width_from_length(self)
- # TODO, should this be the default for everything?
- def copy(self)
Class Vector(Arrow)
class manimlib.mobject.geometry.Vector(Arrow)version 19Dec2019
Configuration
CONFIG = {
"buff": 0,
}
Functions
- def __init__(self, direction=RIGHT, **kwargs)
Class DoubleArrow(Arrow)
class manimlib.mobject.geometry.DoubleArrow(Arrow)version 19Dec2019
Functions
- def __init__(self, *args, **kwargs)
Class CubicBezier(VMobject)
class manimlib.mobject.geometry.CubicBezier(VMobject)version 19Dec2019
Functions
- def __init__(self, points, **kwargs)
Class Polygon(VMobject)
class manimlib.mobject.geometry.Polygon(VMobject)version 19Dec2019
Configuration
CONFIG = {
"color": BLUE,
}
Functions
- def __init__(self, *vertices, **kwargs)
- def get_vertices(self)
- def round_corners(self, radius=0.5)
Class RegularPolygon(Polygon)
class manimlib.mobject.geometry.RegularPolygon(Polygon)version 19Dec2019
Configuration
CONFIG = {
"start_angle": None,
}
Functions
- def __init__(self, n=6, **kwargs)
Class Triangle(RegularPolygon)
class manimlib.mobject.geometry.Triangle(RegularPolygon)version 19Dec2019
Functions
- def __init__(self, **kwargs)
Class ArrowTip(Triangle)
class manimlib.mobject.geometry.ArrowTip(Triangle)version 19Dec2019
Configuration
CONFIG = {
"fill_opacity": 1,
"stroke_width": 0,
"length": DEFAULT_ARROW_TIP_LENGTH,
"start_angle": PI,
}
Functions
- def __init__(self, **kwargs)
- def get_base(self)
- def get_tip_point(self)
- def get_vector(self)
- def get_angle(self)
- def get_length(self)
Class Rectangle(Polygon)
class manimlib.mobject.geometry.Rectangle(Polygon)version 19Dec2019
Configuration
CONFIG = {
"color": WHITE,
"height": 2.0,
"width": 4.0,
"mark_paths_closed": True,
"close_new_points": True,
}
Functions
- def __init__(self, **kwargs)
Class Square(Rectangle)
class manimlib.mobject.geometry.Square(Rectangle)version 19Dec2019
Configuration
CONFIG = {
"side_length": 2.0,
}
Functions
- def __init__(self, **kwargs)
Class RoundedRectangle(Rectangle)
class manimlib.mobject.geometry.RoundedRectangle(Rectangle)version 19Dec2019
Configuration
CONFIG = {
"corner_radius": 0.5,
}
Functions
- def __init__(self, **kwargs)
Source and Reference
https://github.com/3b1b/manim 19Dec2019
©sideway
ID: 200402302 Last Updated: 4/23/2020 Revision: 0
|
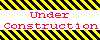 |